This post was updated on January 13, 2020 to adopt the server-side registration approach described in the Block Editor handbook.
A few years ago, Matt Mullenweg urged us all to “Learn JavaScript Deeply.” Becoming more familiar and comfortable with JavaScript is increasingly important for those of us who build the web. In the world of WordPress, projects like Gutenberg have begun taking a major step into a JavaScript-powered future.
For designers and developers who are still getting used to these new languages and workflows, creating and customizing Gutenberg blocks can be somewhat intimidating. There are excellent toolkits like Create GutenBlock to help you get started, but even using those, building a block requires quite a bit of JavaScript familiarity. You also have to make sure you’ve got the right version of Node, learn some terminal commands, install some dependencies, etc. There’s quite a bit of up-front work necessary before you can jump in and start actually building something.
Despite these hurdles, we’ve seen an inspiring number of new block plugins surface lately: CoBlocks, and Atomic Blocks are great examples. They each add a variety of rich blocks, and truly enhance the site-building experience.
There’s a much simpler way to start customizing Gutenberg blocks though, and it’s something we haven’t seen utilized much at this point: block styles. They take only a few minutes to pick up, and mostly just require you to know CSS.
You’ve likely noticed block styles while using Gutenberg. The Quote, Pullquote, Separator, Table, and Button blocks ship with some alternate styles by default.
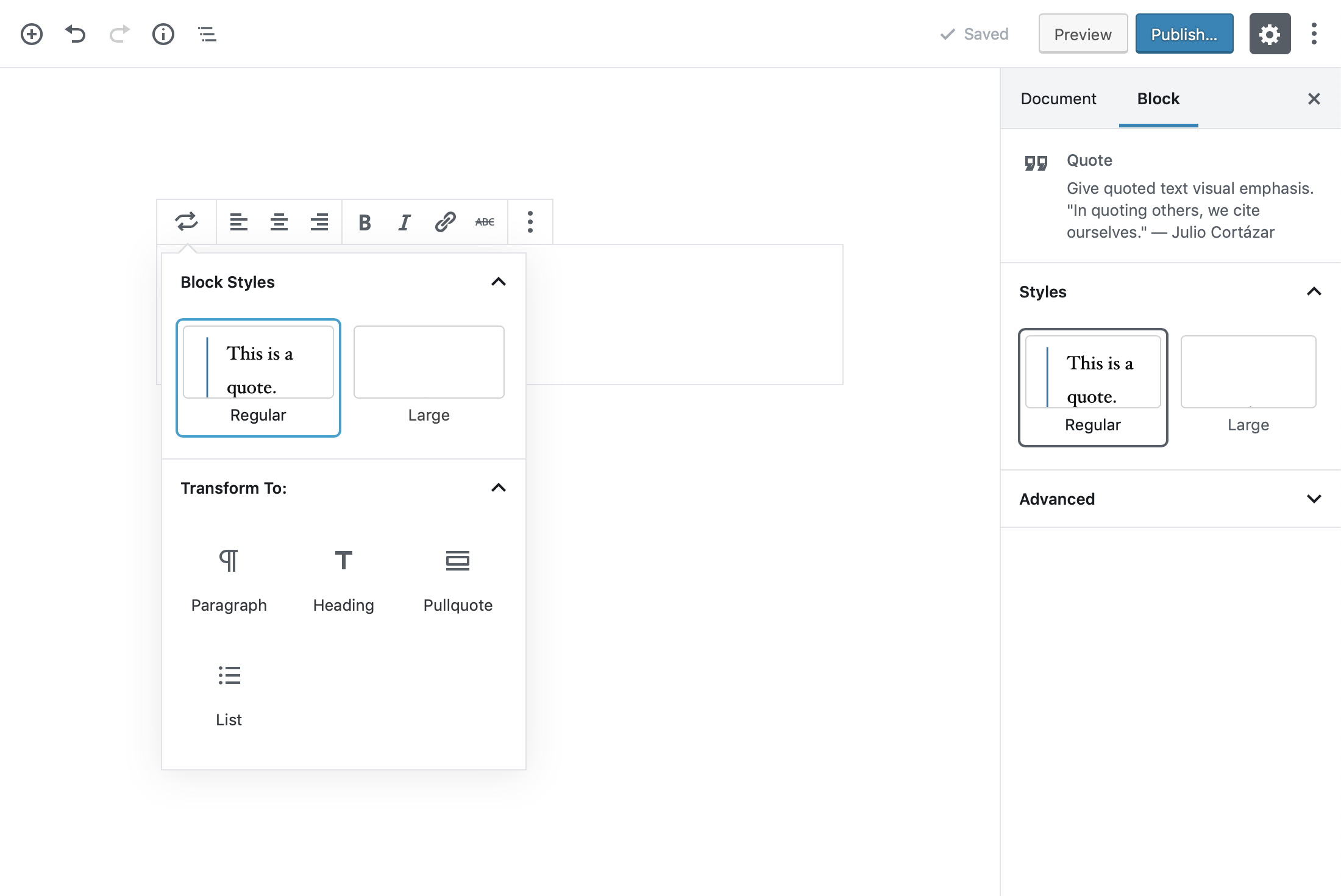
Block styles are an excellent but relatively unused area of Gutenberg customization. They’re a simple way to get started with Gutenberg development: With a few lines of CSS, you can build something that feels like a whole new custom block. Also, since they’re reusable across multiple sites, block styles help pave the way for a more flexible system of components that make up a site’s theme.
Technically, block styles are very simple. They’re composed of two things:
- A few lines of PHP (or JavaScript) to declare the block variation and give it a custom class name.
- CSS styles to fine-tune the appearance.
That’s it. A block style is a custom block class, with some styles applied.
Implementation
A block style can be implemented in a plugin or in a theme. For this example, I’m going to focus on making a plugin. One of the benefits of using a plugin is that it’s not tied to the user’s theme. This allows your block style to be used across any site, running any theme — as if you had built an entirely new custom block.
Block styles can be defined in JavaScript, or in PHP. To keep things simple, we’re going to focus on using the latter. This lets us set everything up with only two files:
index.php
to set up the plugin, declare the block style, and enqueue the stylesheetstyle.css
to house the actual styles
index.php
By default, index.php
just needs to initialize the plugin with a DocBloc, enqueue the CSS file, and register a new block style:
<?php /** * Plugin Name: Block Styles */ /** * Register Custom Block Styles */ if ( function_exists( 'register_block_style' ) ) { function block_styles_register_block_styles() { /** * Register stylesheet */ wp_register_style( 'block-styles-stylesheet', plugins_url( 'style.css', __FILE__ ), array(), '1.1' ); /** * Register block style */ register_block_style( 'core/paragraph', array( 'name' => 'blue-paragraph', 'label' => 'Blue Paragraph', 'style_handle' => 'block-styles-stylesheet', ) ); } add_action( 'init', 'block_styles_register_block_styles' ); }
Let’s take a closer look at the block style registration portion:
register_block_style( 'core/paragraph', array( 'name' => 'blue-paragraph', 'label' => 'Blue Paragraph', 'style_handle' => 'block-styles-stylesheet', ) );
The second line tells Gutenberg which existing block to start with. In this example, we are going to create a block style for the Paragraph block. Any existing block can inherit a block style, so if you were to target a different block, you’d change core/paragraph
to the name of a different block. Technically, these names are found in the Gutenberg block source code, but they’re usually easily guessable. For example: core/cover
, core/separator
, etc.
The name
parameter on the fourth line creates the custom class that’ll be assigned to the block. In this case, I’m calling it a blue-paragraph
, but it can be anything you’d like. Since this will be used for a CSS class, be sure not to use any spaces.
The label
parameter corresponds to the text label for your block style. This will be shown in the interface when someone opens the “Block Styles” panel.
Finally, style_handle
points WordPress to the stylesheet where it can find the CSS for your block style.
style.css
The CSS file is pretty straightforward. Use the custom class name you created in block.js
(along with a is-style-
prefix), and insert your styles inside:
.is-style-blue-paragraph {
// Custom CSS styles go here.
}
Your styles here will build off of the default block styles, and will be loaded in before a theme’s editor styles. So be sure to keep your selectors specific enough to override those potentially competing styles.
Block Styles Plugin Boilerplate
To help you get started, I’ve created a GitHub repository that you can clone and use to get started. It contains the three files detailed above, along with some light documentation.
https://github.com/Automattic/gutenberg-block-styles
Out of the box, the repository is a small plugin that adds a “Blue Paragraph” block style to the Paragraph block:
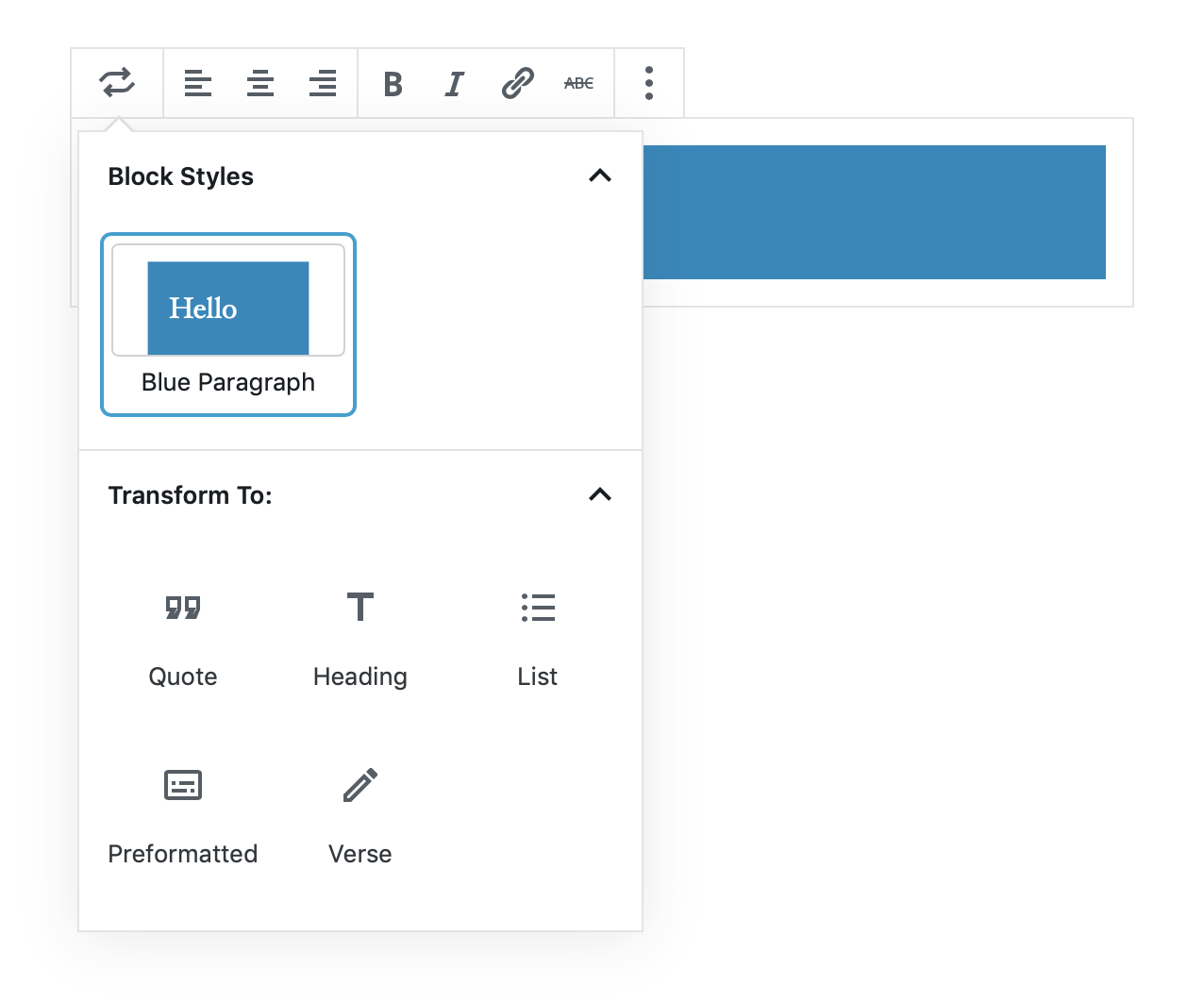
With a few small adjustments, it’s possible to change this into something drastically different. There’s another branch on that GitHub repository that includes a Music theme-style cover block variant:
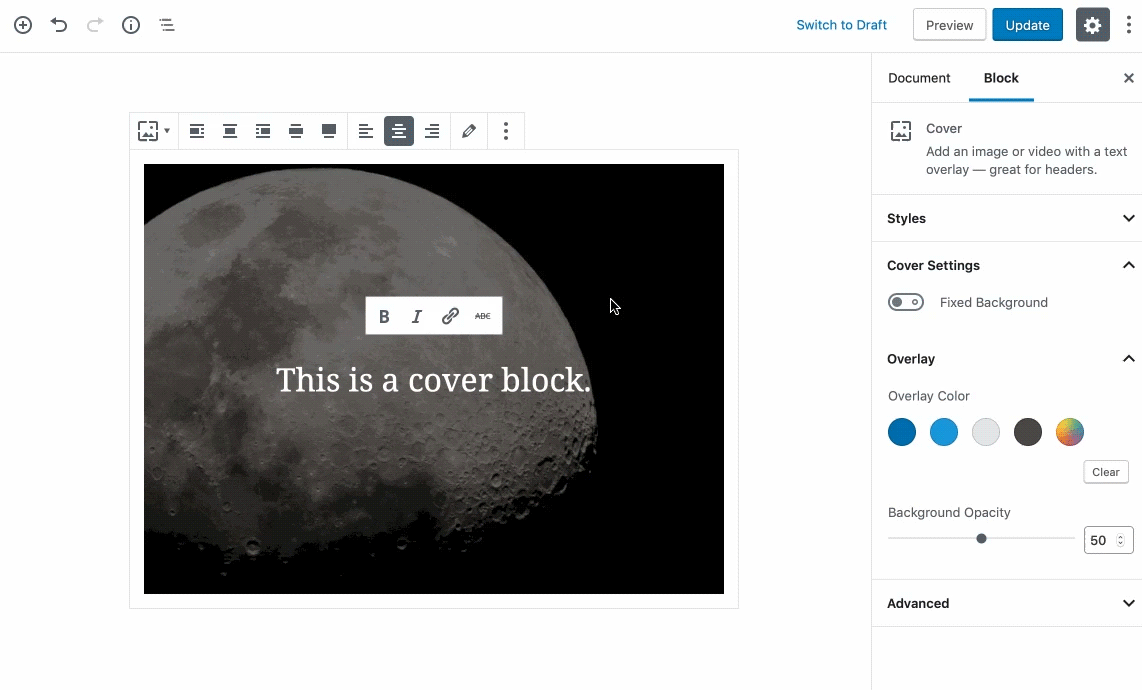
I’ve included a third branch that adds a somewhat ridiculous animation to the text (or emoji) inside of a Cover block:
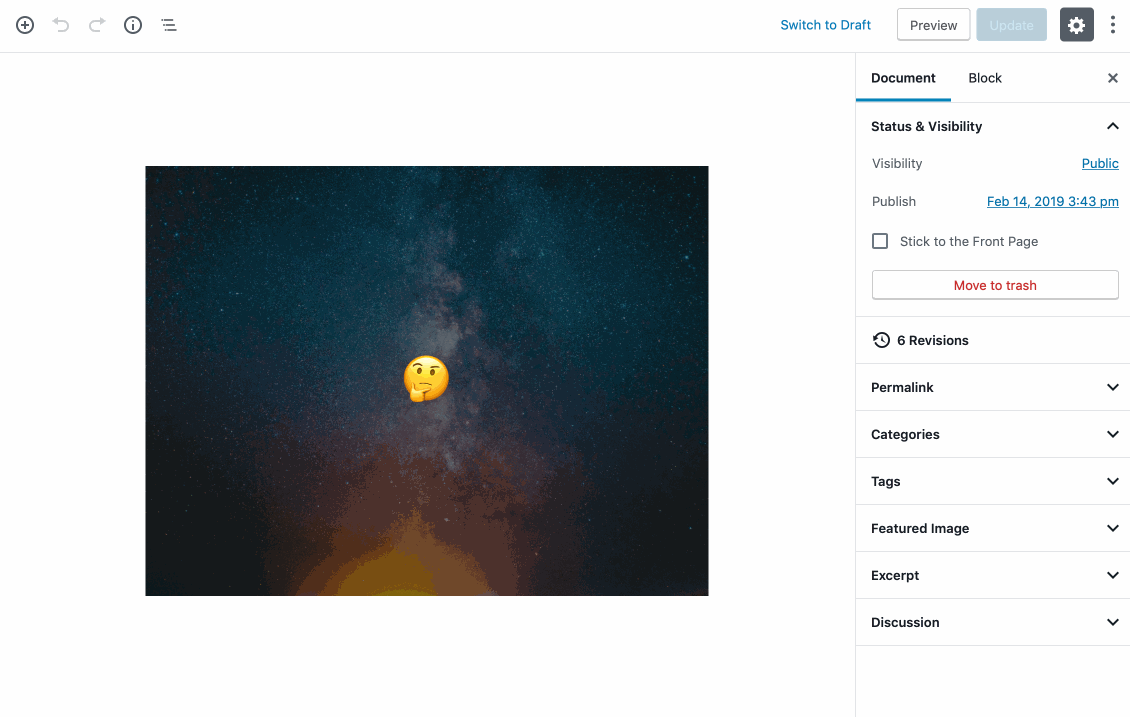
Both of these are accomplished by changing about a dozen lines of code in the repo. They took minutes to create, and work across any site that uses Gutenberg, regardless of the theme it’s using.
What will you create?
Block styles are ripe with possibility. I’d love to see a CSS Zen Garden-style plugin someday that showcases dozens of different ways to customize blocks through block styles.
In the meantime, have you built something cool using block styles? If so, I’d love to hear about it. Please share your explorations in the comments.
2 responses
Block variations is really powerful. With a single CSS class, we can make a lot of things with just CSS. I’ve seen that a lot with simple themes on WordPress.com. CSS is more powerful than we think.
Just a question: are block variations allowed in themes on wordpress.org, or they have to be in plugins?
They are allowed!
In fact, the theme review team recently added a new “Block Editor Styles” tag to the WordPress.org repo, so you can filter by themes that include them.EDIT: After further discussion with the theme review folks, the block-styles tag is actually intended for just general block styles (as in: the theme provides styles for all the default Gutenberg blocks). But Block Styles should be allowed under their current guidelines. (WP.org slack discussion)